In this example we connect an Ethernet shield to an Arduino, we then connect a MPL3115A2 sensor to this and we will send the values to Thingspeak
Lets take a look at the MPL3115A2.
Sensor Overview
The MPL3115A2 is a compact, piezoresistive, absolute pressure sensor with an I2C digital interface. MPL3115A2 has a wide operating range of 20 kPa to 110 kPa, a range that covers all surface elevations on earth. The MEMS is temperature compensated utilizing an on-chip temperature sensor. The pressure and temperature data is fed into a high resolution ADC to provide fully compensated and digitized outputs for pressure in Pascals and temperature in °C.
The compensated pressure output can then be converted to altitude, utilizing the formula stated in Section 9.1.3 “Pressure/altitude” provided in meters.The internal processing in MPL3115A2 removes compensation and unit conversion load from the system MCU, simplifying system design
Thingspeak setup
You will now need to create a new account at thingspeak – https://thingspeak.com. Once done create a new channel and add one new field called temperature. You can see this in a screen capture of my simple channel, notice the ChannelID you will need that in your code later. You can also fill in other fields such as Name, description and there are a few others as well. The key one(s) are Field1, Field 2 and Field 3 – this effectively is the data you send to thingspeak
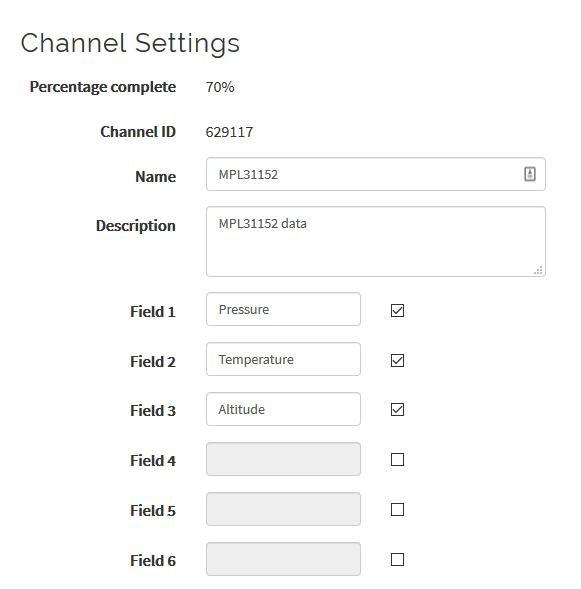
Parts List
Part | Link |
Arduino Uno | UNO R3 CH340G/ATmega328P, compatible for Arduino UNO |
MPL3115A2 module | MPL3115A2 I2C Intelligent Temperature Pressure Altitude Sensor V2.0 for Arduino |
Connecting cable | Free shipping Dupont line 120pcs 20cm male to male + male to female and female to female jumper wire |
Arduino Ethernet shield | Ethernet Shield W5100 R3 UNO and Mega 2560 |
Schematics/Layout
Connect the Ethernet shield and the connect the sensor to the shield connector, like this.
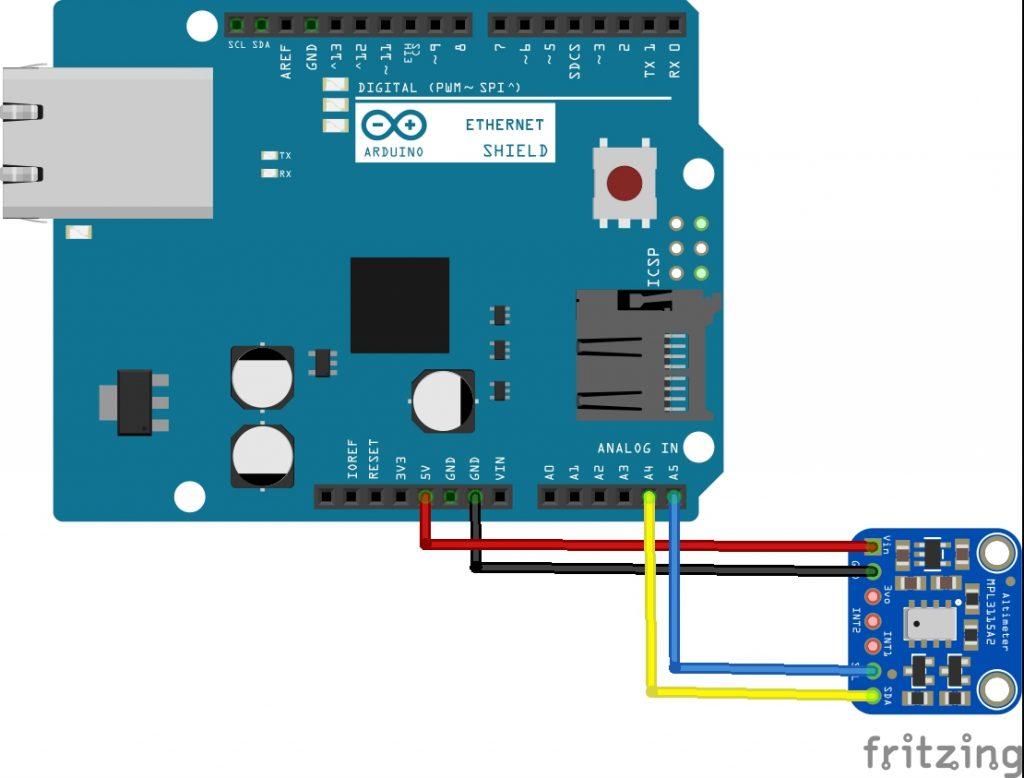
Code
Again we use a library for the sensor, the rest are built in libraries – https://github.com/adafruit/Adafruit_MPL3115A2_Library
You will also need the thingspeak library – https://github.com/mathworks/thingspeak-arduino
[codesyntax lang=”cpp”]
#define USE_ETHERNET_SHIELD #include "ThingSpeak.h" #include <Wire.h> #include <Adafruit_MPL3115A2.h> #include <SPI.h> #include <Ethernet.h> byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED}; EthernetClient client; Adafruit_MPL3115A2 baro = Adafruit_MPL3115A2(); //you will get these from thingspeak unsigned long myChannelNumber = channelhere; const char * myWriteAPIKey = "apikeyhere"; void setup() { //start the serial Serial.begin(9600); //network stuff Ethernet.begin(mac); ThingSpeak.begin(client); //start the sensor if (! baro.begin()) { Serial.println("Couldnt find sensor"); return; } } void loop() { float pascals = baro.getPressure(); float altm = baro.getAltitude(); float tempC = baro.getTemperature(); // Write to ThingSpeak. There are up to 8 fields in a channel, allowing you to store up to 8 different // pieces of information in a channel. ThingSpeak.setField(1, pascals/3377); ThingSpeak.setField(2, tempC); ThingSpeak.setField(3, altm); int x = ThingSpeak.writeFields(myChannelNumber, myWriteAPIKey); if(x == 200) { Serial.println("Channel update successful."); } else { Serial.println("Problem updating channel. HTTP error code " + String(x)); } delay(20000); // ThingSpeak will only accept updates every 15 seconds. }
[/codesyntax]
Output
Open your channel and you see data starting to appear – this was my channel data
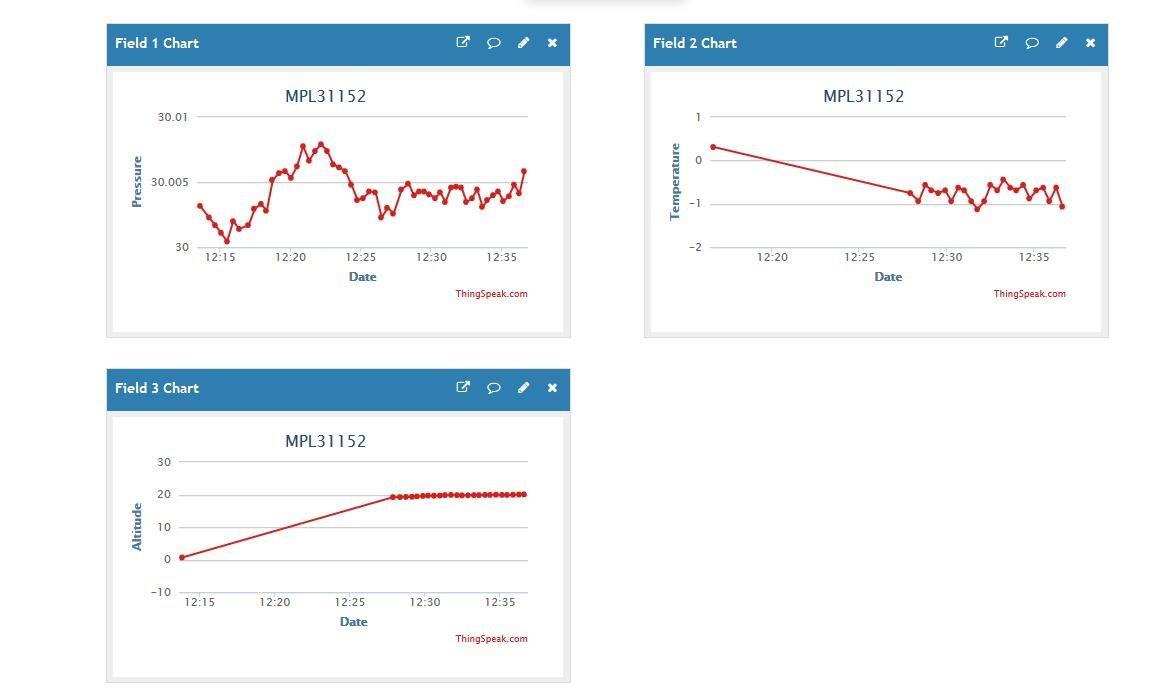
Links
https://www.nxp.com/docs/en/data-sheet/MPL3115A2.pdf