In this article we look at an TCS34725 connected to a Beaglebone first of all lets look at the sensor.
The TCS34725 device provides a digital return of red, green, blue (RGB), and clear light sensing values. An IR blocking filter, integrated on-chip and localized to the color sensing photodiodes, minimizes the IR spectral component of the incoming light and allows color measurements to be made accurately.
The high sensitivity, wide dynamic range, and IR blocking filter make the TCS34725 an ideal color sensor solution for use under varying lighting conditions and through attenuating materials. This data is transferred via an I2C to the host.
Features
- Integrated IR blocking filter
- 3.8M:1 dynamic range
- Four independent analog-to-digital converters
- A reference channel for color analysis (clear channel photodiode)
Benefits
- Minimizes IR and UV spectral component effects to produce accurate color measurement
- Enables accurate color and ambient light sensing under varying lighting conditions
- Minimizes motion/transient errors
- Clear channel provides a reference which allows for isolation of color content
Parts Required
Schematic/Connection
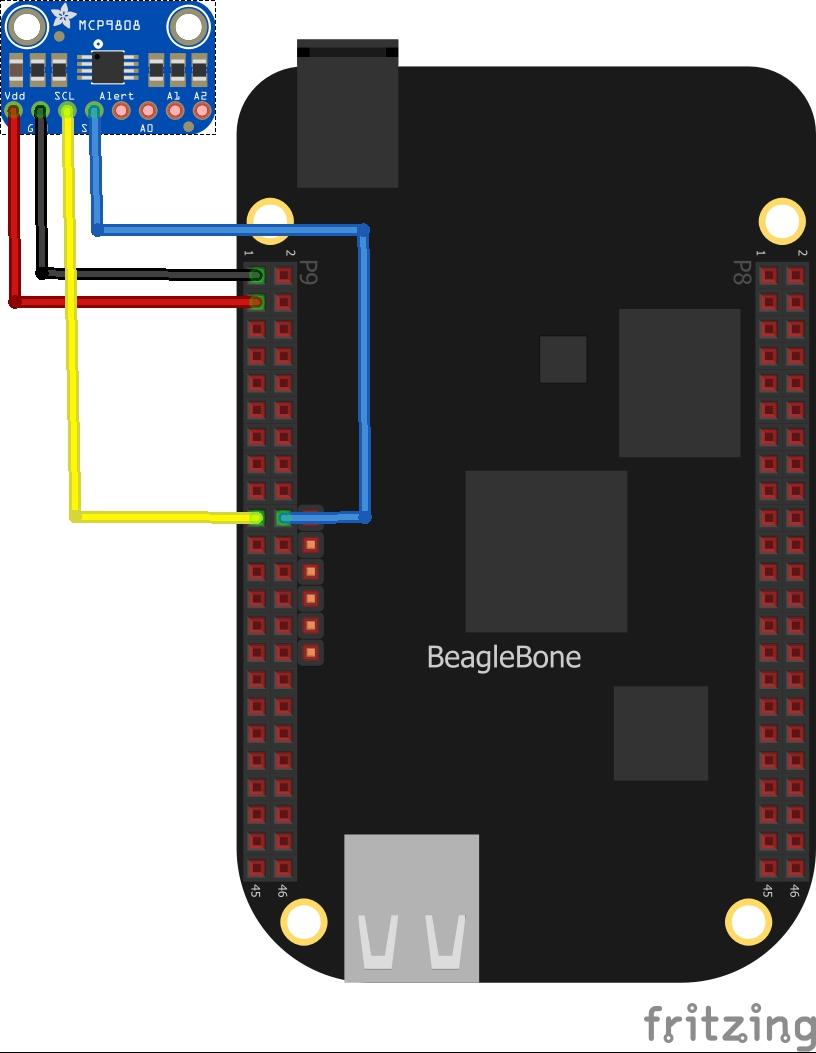
Code Example
This is a controleverything example – they have code examples for various platforms. This is the C example from https://github.com/ControlEverythingCommunity/TCS34725
[codesyntax lang=”cpp”]
#include <stdio.h> #include <stdlib.h> #include <linux/i2c-dev.h> #include <sys/ioctl.h> #include <fcntl.h> void main() { // Create I2C bus int file; char *bus = "/dev/i2c-2"; if ((file = open(bus, O_RDWR)) < 0) { printf("Failed to open the bus. \n"); exit(1); } // Get I2C device, TCS34725 I2C address is 0x29(41) ioctl(file, I2C_SLAVE, 0x29); // Select enable register(0x80) // Power ON, RGBC enable, wait time disable(0x03) char config[2] = {0}; config[0] = 0x80; config[1] = 0x03; write(file, config, 2); // Select ALS time register(0x81) // Atime = 700 ms(0x00) config[0] = 0x81; config[1] = 0x00; write(file, config, 2); // Select Wait Time register(0x83) // WTIME : 2.4ms(0xFF) config[0] = 0x83; config[1] = 0xFF; write(file, config, 2); // Select control register(0x8F) // AGAIN = 1x(0x00) config[0] = 0x8F; config[1] = 0x00; write(file, config, 2); sleep(1); // Read 8 bytes of data from register(0x94) // cData lsb, cData msb, red lsb, red msb, green lsb, green msb, blue lsb, blue msb char reg[1] = {0x94}; write(file, reg, 1); char data[8] = {0}; if(read(file, data, 8) != 8) { printf("Erorr : Input/output Erorr \n"); } else { // Convert the data int cData = (data[1] * 256 + data[0]); int red = (data[3] * 256 + data[2]); int green = (data[5] * 256 + data[4]); int blue = (data[7] * 256 + data[6]); // Calculate luminance float luminance = (-0.32466) * (red) + (1.57837) * (green) + (-0.73191) * (blue); if(luminance < 0) { luminance = 0; } // Output data to screen printf("Red color luminance : %d lux \n", red); printf("Green color luminance : %d lux \n", green); printf("Blue color luminance : %d lux \n", blue); printf("IR luminance : %d lux \n", cData); printf("Ambient Light Luminance : %.2f lux \n", luminance); } }
[/codesyntax]
Save this as TCS34725.c, I used the Cloud 9 IDE
First of all compile the c program.
$>gcc TCS34725.c -o TCS34725
Run the c program.
$>./TCS34725
Output
After running you should see something like this
Red color luminance : 1891 lux
Green color luminance : 1465 lux
Blue color luminance : 1382 lux
IR luminance : 2471 lux
Ambient Light Luminance : 686.88 lux