In this example we take a look at an MLX90615 IR thermometer, we will connect this to a LinkIT one. There will be 2 examples to look at which are written using the Arduino IDE
The MLX90615 is a miniature infrared thermometer for non-contact temperature measurements. Both the IR sensitive thermopile detector chip and the signal conditioning ASIC are integrated in the same miniature TO-46 can.
The infrared thermometer comes factory calibrated with a digital SMBus output giving full access to the measured temperature in the complete temperature range(s) with a resolution of 0.02 °C. The sensor achieves an accuracy of ±0.2°C within the relevant medical temperature range. The user can choose to configure the digital output to be PWM.
Features and benefits
Factory calibrated in wide temperature range: -20 to 85°C for sensor temperature and -40 to 115°C for object temperature
High accuracy of 0.5°C over wide temperature range (0..+50 C for both Ta and To)
Medical accuracy of 0.2°C in a limited temperature range
Measurement resolution of 0.02°C
SMBus compatible digital interface for fast temperature readings and building sensor networks
Customizable PWM output for continuous reading
3V supply voltage with power saving mode
Parts List
Amount | Part Type |
---|---|
1 | MLX90615 Digital Infrared Temperature Sensor for Arduino GY Series Module |
1 | LinkIt ONE MT2502A wireless 2503 development board |
Connection
An easy device to connect, simple I2C sensor that requires 3.3v and GND.
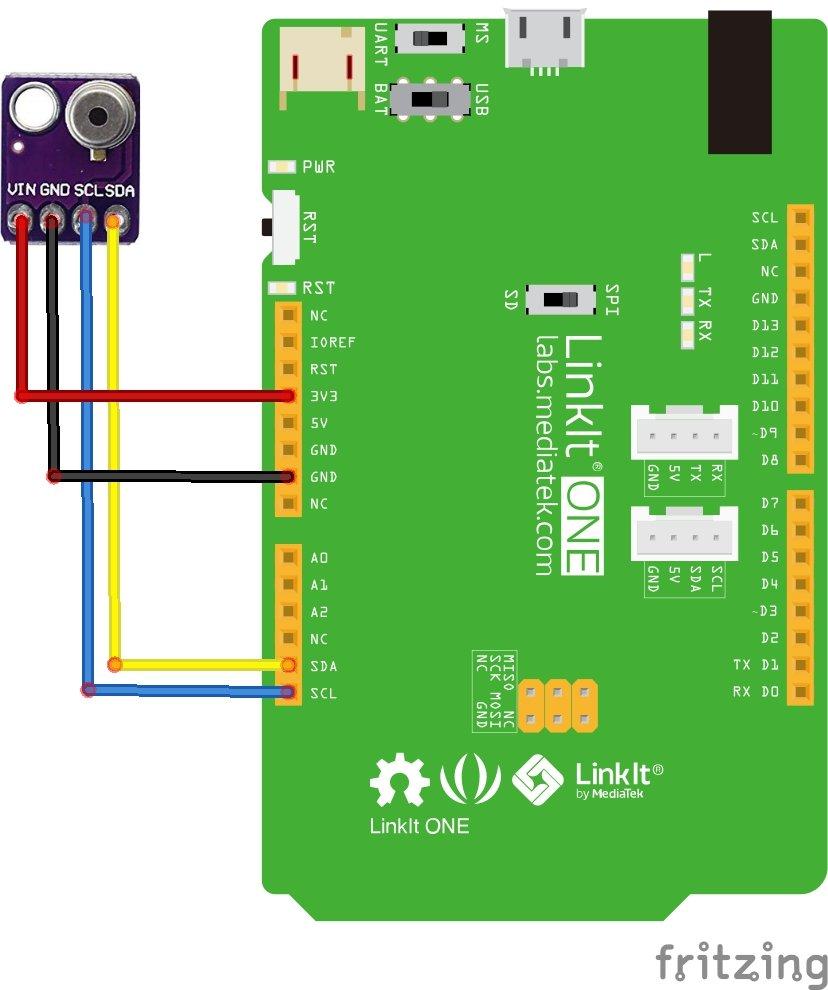
Test Code
This particular example comes from the following library which I installed – https://github.com/skiselev/MLX90615
[codesyntax lang=”cpp”]
#include <Wire.h> #include <mlx90615.h> MLX90615 mlx = MLX90615(); void setup() { Serial.begin(9600); Serial.println("Melexis MLX90615 infra-red temperature sensor test"); mlx.begin(); Serial.print("Sensor ID number = "); Serial.println(mlx.get_id(), HEX); } void loop() { Serial.print("Ambient = "); Serial.print(mlx.get_ambient_temp()); Serial.print(" *C\tObject = "); Serial.print(mlx.get_object_temp()); Serial.println(" *C"); delay(500); }
[/codesyntax]
Test Output
In the serial monitor and you should see something like this. The warmer object reading was when I placed my finger near the sensor
Ambient = 24.31 *C Object = 33.23 *C
Ambient = 24.23 *C Object = 31.63 *C
Ambient = 24.19 *C Object = 32.25 *C
Ambient = 24.23 *C Object = 37.57 *C
Ambient = 24.27 *C Object = 38.31 *C
Ambient = 24.37 *C Object = 37.11 *C
Ambient = 24.41 *C Object = 36.87 *C
Ambient = 24.45 *C Object = 35.99 *C
Ambient = 24.49 *C Object = 35.47 *C
Ambient = 24.49 *C Object = 33.81 *C
Web page Example
There are 3 lines that will/may need changed for your Wifi details
#define WIFI_AP "wifi ssid here" #define WIFI_PASSWORD "password here" #define WIFI_AUTH LWIFI_WPA The last one you can select from from LWIFI_OPEN, LWIFI_WPA, or LWIFI_WEP according to your WiFi AP configuration
Now for the complete code example
[codesyntax lang=”cpp”]
#include <LTask.h> #include <LWiFi.h> #include <LWiFiServer.h> #include <LWiFiClient.h> #include <mlx90615.h> #define WIFI_AP "iainhendry" #define WIFI_PASSWORD "iain061271" #define WIFI_AUTH LWIFI_WPA MLX90615 mlx = MLX90615(); LWiFiServer server(80); void setup() { LTask.begin(); LWiFi.begin(); Serial.begin(9600); //output MLX90615 info via serial Serial.println("Melexis MLX90615 infra-red temperature sensor test"); mlx.begin(); Serial.print("Sensor ID number = "); Serial.println(mlx.get_id(), HEX); // keep retrying until connected to AP Serial.println("Connecting to AP"); while (0 == LWiFi.connect(WIFI_AP, LWiFiLoginInfo(WIFI_AUTH, WIFI_PASSWORD))) { delay(1000); } //connect and display IP address printWifiStatus(); Serial.println("Start Server"); server.begin(); Serial.println("Server Started"); } int loopCount = 0; void loop() { // put your main code here, to run repeatedly: delay(500); loopCount++; LWiFiClient client = server.available(); if (client) { Serial.println("new client"); // an http request ends with a blank line boolean currentLineIsBlank = true; unsigned long timer_out = millis(); while (client.connected()) { if(millis()-timer_out > 5000)break; if (client.available()) { // we basically ignores client request, but wait for HTTP request end int c = client.read(); Serial.print((char)c); if (c == '\n' && currentLineIsBlank) { Serial.println("send response"); // send a standard http response header client.println("HTTP/1.1 200 OK"); client.println("Content-Type: text/html"); client.println("Connection: close"); // the connection will be closed after completion of the response client.println("Refresh: 5"); // refresh the page automatically every 5 sec client.println(); client.println("<!DOCTYPE HTML>"); client.println("<html>"); // output the value of each analog input pin client.print("Ambient = "); client.print(mlx.get_ambient_temp()); client.print(" *C\tObject = "); client.print(mlx.get_object_temp()); client.println(" *C"); break; } if (c == '\n') { // you're starting a new line currentLineIsBlank = true; } else if (c != '\r') { // you've gotten a character on the current line currentLineIsBlank = false; } } } // give the web browser time to receive the data delay(500); // close the connection: Serial.println("close connection"); client.stop(); Serial.println("client disconnected"); } } void printWifiStatus() { // print the SSID of the network you're attached to: Serial.print("SSID: "); Serial.println(LWiFi.SSID()); // print your WiFi shield's IP address: IPAddress ip = LWiFi.localIP(); Serial.println("Please open your browser, and input the following address:"); Serial.println(ip); Serial.print("\r\nsubnet mask: "); Serial.println(LWiFi.subnetMask()); Serial.print("gateway IP: "); Serial.println(LWiFi.gatewayIP()); // print the received signal strength: long rssi = LWiFi.RSSI(); Serial.print("signal strength (RSSI):"); Serial.print(rssi); Serial.println(" dBm"); }
[/codesyntax]
This example has test code to help you out – the printWifiStatus function, open up the USB Modem Port – not the programming port. You should see a message like this
Connecting to AP
SSID: your ssid here
Please open your browser, and input the following address:
192.168.1.15
Web page Output
Open up your favourite web browser and you should see a line that updates every 5 seconds
Ambient = 23.09 *C Object = 24.23 *C
Links
https://www.melexis.com/-/media/files/documents/datasheets/mlx90615-datasheet-melexis.pdf