The LSM303DLHC is a system-in-package featuring a 3D digital linear acceleration sensor and a 3D digital magnetic sensor.
The LSM303DLHC has linear acceleration full scales of ±2g / ±4g / ±8g / ±16g and a magnetic field full scale of ±1.3 / ±1.9 / ±2.5 / ±4.0 / ±4.7 / ±5.6 / ±8.1 gauss.
The LSM303DLHC includes an I2C serial bus interface that supports standard and fast mode 100 kHz and 400 kHz. The system can be configured to generate interrupt signals by inertial wake-up/free-fall events as well as by the positionof the device itself. Thresholds and timing of interrupt generators are programmable by the end user. Magnetic and accelerometer blocks can be enabled or put into power-down mode separately
Features
3 magnetic field channels and 3 acceleration channels
From ±1.3 to ±8.1 gauss magnetic field full scale
±2g/±4g/±8g/±16g linear acceleration full scale
16-bit data output
I2C serial interface
Analog supply voltage 2.16 V to 3.6 V
Power-down mode / low-power mode
2 independent programmable interrupt generators for free-fall and motion detection
Embedded temperature sensor
Embedded FIFO
6D/4D-orientation detection
Parts Required
Connection
Beaglebone | Module |
3.3v – P9.3 | Vcc |
Gnd – P9.1 | Gnd |
SDA – P9.20 | SDA |
SCL – P9.19 | SCL |
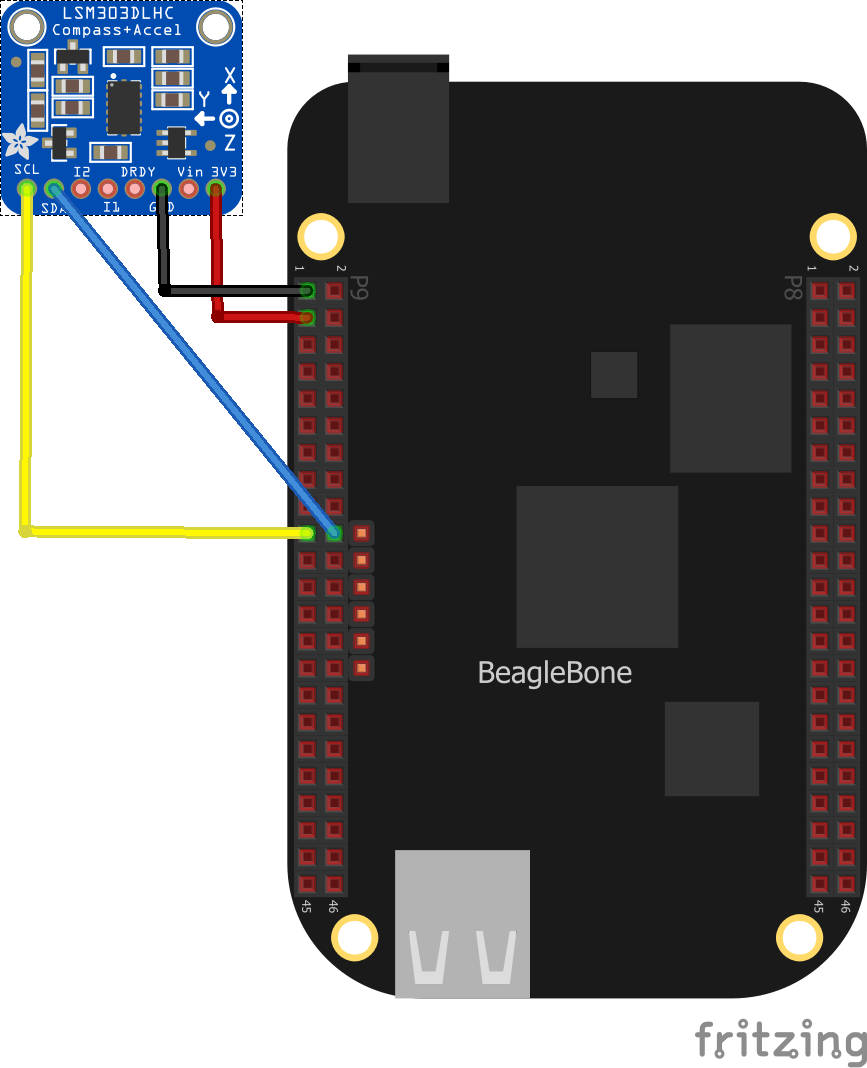
Code
This is a python example, I used the cloud9 IDE
[codesyntax lang=”python”]
# Distributed with a free-will license. # Use it any way you want, profit or free, provided it fits in the licenses of its associated works. # LSM303DLHC # This code is designed to work with the LSM303DLHC_I2CS I2C Mini Module available from ControlEverything.com. # https://www.controleverything.com/products import smbus import time # Get I2C bus bus = smbus.SMBus(2) # LSM303DLHC Accl address, 0x19(25) # Select control register1, 0x20(32) # 0x27(39) Acceleration data rate = 10Hz, Power ON, X, Y, Z axis enabled bus.write_byte_data(0x19, 0x20, 0x27) # LSM303DLHC Accl address, 0x19(25) # Select control register4, 0x23(35) # 0x00(00) Continuos update, Full scale selection = +/-2g, bus.write_byte_data(0x19, 0x23, 0x00) time.sleep(0.5) # LSM303DLHC Accl address, 0x19(25) # Read data back from 0x28(40), 2 bytes # X-Axis Accl LSB, X-Axis Accl MSB data0 = bus.read_byte_data(0x19, 0x28) data1 = bus.read_byte_data(0x19, 0x29) # Convert the data xAccl = data1 * 256 + data0 if xAccl > 32767 : xAccl -= 65536 # LSM303DLHC Accl address, 0x19(25) # Read data back from 0x2A(42), 2 bytes # Y-Axis Accl LSB, Y-Axis Accl MSB data0 = bus.read_byte_data(0x19, 0x2A) data1 = bus.read_byte_data(0x19, 0x2B) # Convert the data yAccl = data1 * 256 + data0 if yAccl > 32767 : yAccl -= 65536 # LSM303DLHC Accl address, 0x19(25) # Read data back from 0x2C(44), 2 bytes # Z-Axis Accl LSB, Z-Axis Accl MSB data0 = bus.read_byte_data(0x19, 0x2C) data1 = bus.read_byte_data(0x19, 0x2D) # Convert the data zAccl = data1 * 256 + data0 if zAccl > 32767 : zAccl -= 65536 # LSM303DLHC Mag address, 0x1E(30) # Select MR register, 0x02(02) # 0x00(00) Continous conversion mode bus.write_byte_data(0x1E, 0x02, 0x00) # LSM303DLHC Mag address, 0x1E(30) # Select CRA register, 0x00(00) # 0x10(16) Temperatuer disabled, Data output rate = 15Hz bus.write_byte_data(0x1E, 0x00, 0x10) # LSM303DLHC Mag address, 0x1E(30) # Select CRB register, 0x01(01) # 0x20(32) Gain setting = +/- 1.3g bus.write_byte_data(0x1E, 0x01, 0x20) time.sleep(0.5) # LSM303DLHC Mag address, 0x1E(30) # Read data back from 0x03(03), 2 bytes # X-Axis Mag MSB, X-Axis Mag LSB data0 = bus.read_byte_data(0x1E, 0x03) data1 = bus.read_byte_data(0x1E, 0x04) # Convert the data xMag = data0 * 256 + data1 if xMag > 32767 : xMag -= 65536 # LSM303DLHC Mag address, 0x1E(30) # Read data back from 0x05(05), 2 bytes # Y-Axis Mag MSB, Y-Axis Mag LSB data0 = bus.read_byte_data(0x1E, 0x07) data1 = bus.read_byte_data(0x1E, 0x08) # Convert the data yMag = data0 * 256 + data1 if yMag > 32767 : yMag -= 65536 # LSM303DLHC Mag address, 0x1E(30) # Read data back from 0x07(07), 2 bytes # Z-Axis Mag MSB, Z-Axis Mag LSB data0 = bus.read_byte_data(0x1E, 0x05) data1 = bus.read_byte_data(0x1E, 0x06) # Convert the data zMag = data0 * 256 + data1 if zMag > 32767 : zMag -= 65536 # Output data to screen print "Acceleration in X-Axis : %d" % xAccl print "Acceleration in Y-Axis : %d" % yAccl print "Acceleration in Z-Axis : %d" % zAccl print "Magnetic field in X-Axis : %d" %xMag print "Magnetic field in Y-Axis : %d" %yMag print "Magnetic field in Z-Axis : %d" %zMag
[/codesyntax]
Output
Here are a couple of runs moving the sensor around
debian@beaglebone:/var/lib/cloud9/$ python LSM303DLHC.py
Acceleration in X-Axis : 3072
Acceleration in Y-Axis : 15872
Acceleration in Z-Axis : -256
Magnetic field in X-Axis : 6
Magnetic field in Y-Axis : -459
Magnetic field in Z-Axis : 237
debian@beaglebone:/var/lib/cloud9/$ python LSM303DLHC.py
Acceleration in X-Axis : -4544
Acceleration in Y-Axis : 960
Acceleration in Z-Axis : 8192
Magnetic field in X-Axis : 83
Magnetic field in Y-Axis : -26
Magnetic field in Z-Axis : 590
Link
https://www.st.com/resource/en/datasheet/DM00027543.pdf