The MLX90614 is a non-contact infrared thermometer with a measurement range from -70 to +380 degree Celsius. Just connect the four leads to your Beaglebone and you will have a accurate thermometer with a resolution of 0.01 and a accuracy of 0.5 degrees, or for that matter you can use any microcontroller that can communicate with it through it’s I2C interface.
Being an I2C device you simply need to connect to the SDA, SCL and choose a suitable GND and Vin.
This version I chose comes with a breakout board with all of the components needed for operation. Here is a picture of that breakout board
Features:
Small size, low cost
Mounted on a breakout board with two types of pins
10k Pull up resistors for the I2C interface with optional solder jumpers
Factory calibrated in wide temperature range:
-40 … + 125 ° C for sensor temperature and
-70 … + 380 ° C for object temperature.
High accuracy of 0.5 ° C over wide temperature range (0 … + 50 ° C for both Ta and To) High (medical) accuracy calibration
Measurement resolution of 0.02 ° C
Single and dual zone versions
SMBus compatible digital interface
Customizable PWM output for continuous reading
Sleep mode for reduced power consumption
Parts List
Connection
I used the following connection from the module above to my Beaglebone
MLX90614 module Connection | Beaglebone Connection |
VIN | P9.3 |
Gnd | P9.1 |
SDA | P9.20 |
SCL | P9.19 |
This is a layout showing the connection
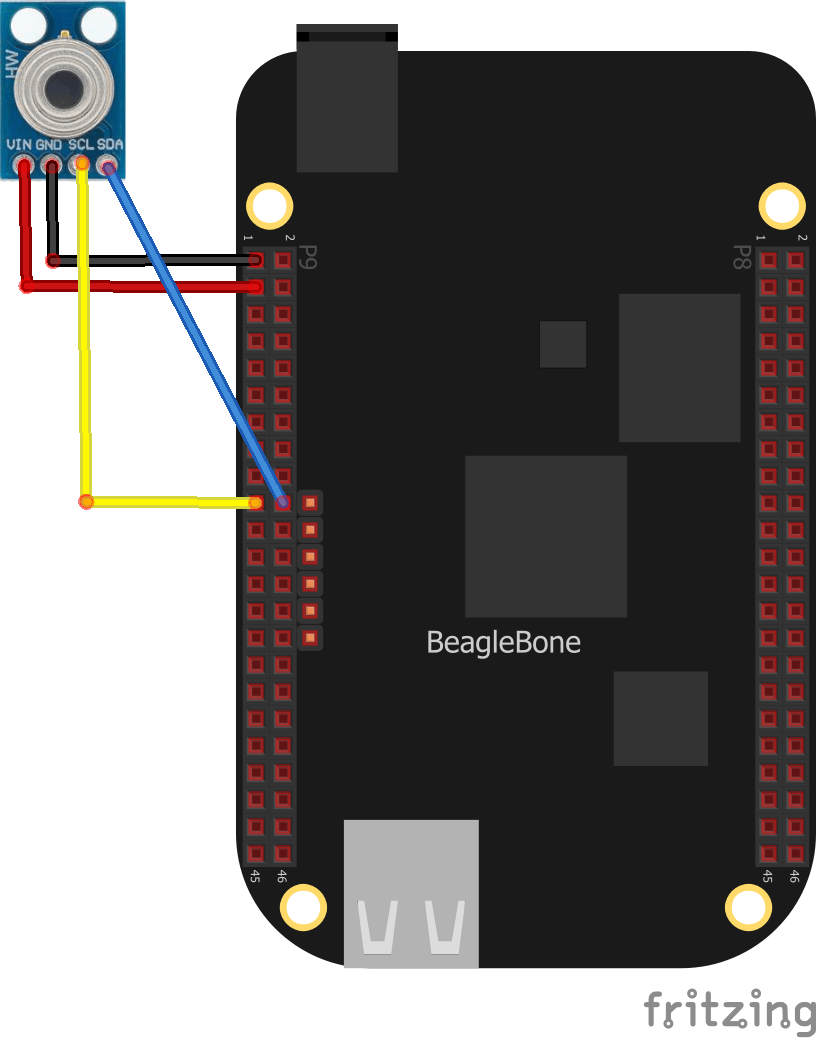
Code
Credit to https://github.com/CRImier/python-MLX90614
You need to save this as mlx90614.py, I used the cloud9 IDE
[codesyntax lang=”python”]
import smbus from time import sleep class MLX90614(): MLX90614_RAWIR1=0x04 MLX90614_RAWIR2=0x05 MLX90614_TA=0x06 MLX90614_TOBJ1=0x07 MLX90614_TOBJ2=0x08 MLX90614_TOMAX=0x20 MLX90614_TOMIN=0x21 MLX90614_PWMCTRL=0x22 MLX90614_TARANGE=0x23 MLX90614_EMISS=0x24 MLX90614_CONFIG=0x25 MLX90614_ADDR=0x0E MLX90614_ID1=0x3C MLX90614_ID2=0x3D MLX90614_ID3=0x3E MLX90614_ID4=0x3F comm_retries = 5 comm_sleep_amount = 0.1 def __init__(self, address=0x5a, bus_num=2): self.bus_num = bus_num self.address = address self.bus = smbus.SMBus(bus=bus_num) def read_reg(self, reg_addr): err = None for i in range(self.comm_retries): try: return self.bus.read_word_data(self.address, reg_addr) except IOError as e: err = e #"Rate limiting" - sleeping to prevent problems with sensor #when requesting data too quickly sleep(self.comm_sleep_amount) #By this time, we made a couple requests and the sensor didn't respond #(judging by the fact we haven't returned from this function yet) #So let's just re-raise the last IOError we got raise err def data_to_temp(self, data): temp = (data*0.02) - 273.15 return temp def get_amb_temp(self): data = self.read_reg(self.MLX90614_TA) return self.data_to_temp(data) def get_obj_temp(self): data = self.read_reg(self.MLX90614_TOBJ1) return self.data_to_temp(data) if __name__ == "__main__": sensor = MLX90614() print(sensor.get_amb_temp()) print(sensor.get_obj_temp())
[/codesyntax]
Now create another file called test_mlx90614.py and entering the following
[codesyntax lang=”python”]
from mlx90614 import MLX90614 thermometer_address = 0x5a thermometer = MLX90614(thermometer_address) print thermometer.get_amb_temp() print thermometer.get_obj_temp()
[/codesyntax]
Output
Open a terminal in the Cloud9 IDE and enter python test_mlx90614.py
Here you can see a couple of test runs
debian@beaglebone:/var/lib/cloud9/iain/MLX90614$ python test_mlx90614.py 21.67 57.85 debian@beaglebone:/var/lib/cloud9/iain/MLX90614$ python test_mlx90614.py 22.41 54.79 debian@beaglebone:/var/lib/cloud9/iain/MLX90614$ python test_mlx90614.py 23.11 24.59 debian@beaglebone:/var/lib/cloud9/iain/MLX90614$ python test_mlx90614.py 22.69 26.09