In this example we look at the MMA7660FC accelerometer connected to a Beaglebone
The MMA7660FC is a digital output I²C, very low-power, low-profile capacitive micro-machined accelerometer featuring a low pass filter, compensation for zero-g offset and gain errors and conversion to six-bit digital values at a user configurable output data rate. The device can be used for sensor data changes, product orientation and gesture detection through an interrupt pin (INT).
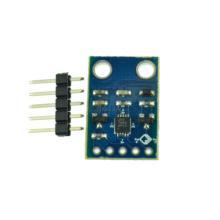
- Digital output I²C
- 3 mm x 3 mm x 0.9 mm DFN package
- Low-power current consumption
- Off mode: 0.4 µA
- Standby mode: 2 µA
- Active mode: Configurable down to 47 µA
- Low-voltage operation: 2.4 – 3.6-volts
- 3-axis ±1.5 g MEMS sensor and CMOS interface controller built into one package
- Configurable output data rate from one to 120 samples a second
- Auto wake/sleep feature for low-power consumption
- Tilt orientation detection for portrait/landscape capability
- Gesture detection including shake and pulse detection
Parts List
Connection
Beaglebone |
MMA7660 |
3v3 – P9.3 |
Vcc |
Gnd – P9.1 |
Gnd |
SDA – P9.210 |
SDA |
SCL – P9.19 |
SCL |
Code
This is an example from controleverything called MMA7660FC.py
[codesyntax lang=”python”]
import smbus
import time
# Get I2C bus
bus = smbus.SMBus(2)
# MMA7660FC address, 0x4C(76)
# Select mode register, 0x07(07)
# 0x01(01) Active mode
bus.write_byte_data(0x4C, 0x07, 0x01)
# MMA7660FC address, 0x4C(76)
# Select sample rate register, 0x08(08)
# 0x07(07) 1 Sample/second active
bus.write_byte_data(0x4C, 0x08, 0x07)
time.sleep(0.5)
# MMA7660FC address, 0x4C
# Read data back from 0x00, 3 bytes
# X-Axis Accl, Y-Axis Accl, Z-Axis Accl
data=bus.read_i2c_block_data(0x4C, 0x00, 3)
# Convert the data to 6-bits
xAccl = data[0] & 0x3F
if xAccl > 31 :
xAccl -= 64
yAccl = data[1] & 0x3F
if yAccl > 31 :
yAccl -= 64
zAccl = data[2] & 0x3F
if zAccl > 31 :
zAccl -= 64
# Output data to screen
print "Acceleration in X-Axis : %d" %xAccl
print "Acceleration in Y-Axis : %d" %yAccl
print "Acceleration in Z-Axis : %d" %zAccl
[/codesyntax]
Output
run this by typing in
debian@beaglebone:/var/lib/cloud9/$ python mma7660.py
Acceleration in X-Axis : -10
Acceleration in Y-Axis : -19
Acceleration in Z-Axis : 0
debian@beaglebone:/var/lib/cloud9/$ python mma7660.py
Acceleration in X-Axis : -15
Acceleration in Y-Axis : -16
Acceleration in Z-Axis : -14
debian@beaglebone:/var/lib/cloud9/$ python mma7660.py
Acceleration in X-Axis : -20
Acceleration in Y-Axis : -4
Acceleration in Z-Axis : 15
debian@beaglebone:/var/lib/cloud9/$
Link
https://www.nxp.com/docs/en/data-sheet/MMA7660FC.pdf