In this article we look at another sensor – this time its the TMD2771 and we will connect it to our Beaglebone and we will have a python example
First lets take a look at the sensor in question
Ambient Light Sensing, Proximity Detection, and IR LED in a Single Optical Module
Ambient Light Sensing (ALS)
—-Approximates Human Eye Response
—-Programmable Analog Gain
—-Programmable Integration Time
—-Programmable Interrupt Function with Upper and Lower Threshold
—-Up to 16 Bits Resolution
—-Very High Sensitivity – Operates Behind Darkened Glass
—-Up to 1,000,000:1 Dynamic Range
Proximity Detection
—-Calibrated to 100mm Detection
—-Eliminates Factory Calibration of Prox
—-Programmable Number of IR Pulses
—-Programmable Current Sink for the IR LED – No Limiting Resistor Needed
—-Programmable Interrupt Function with Upper and Lower ThresholdD
Programmable Wait Timer
—-Wait State – 65mA Typical Current
—-Programmable from 2.72ms to >8 Seconds
—-I2C Interface Compatible Up to 400 kHz (I2C Fast Mode)
—-Dedicated Interrupt Pin
—-3.94 mm y 2.4 mm y 1.35 mm Package
—-Sleep Mode – 2.5 mA Typical
Parts Required
Schematic/Connection
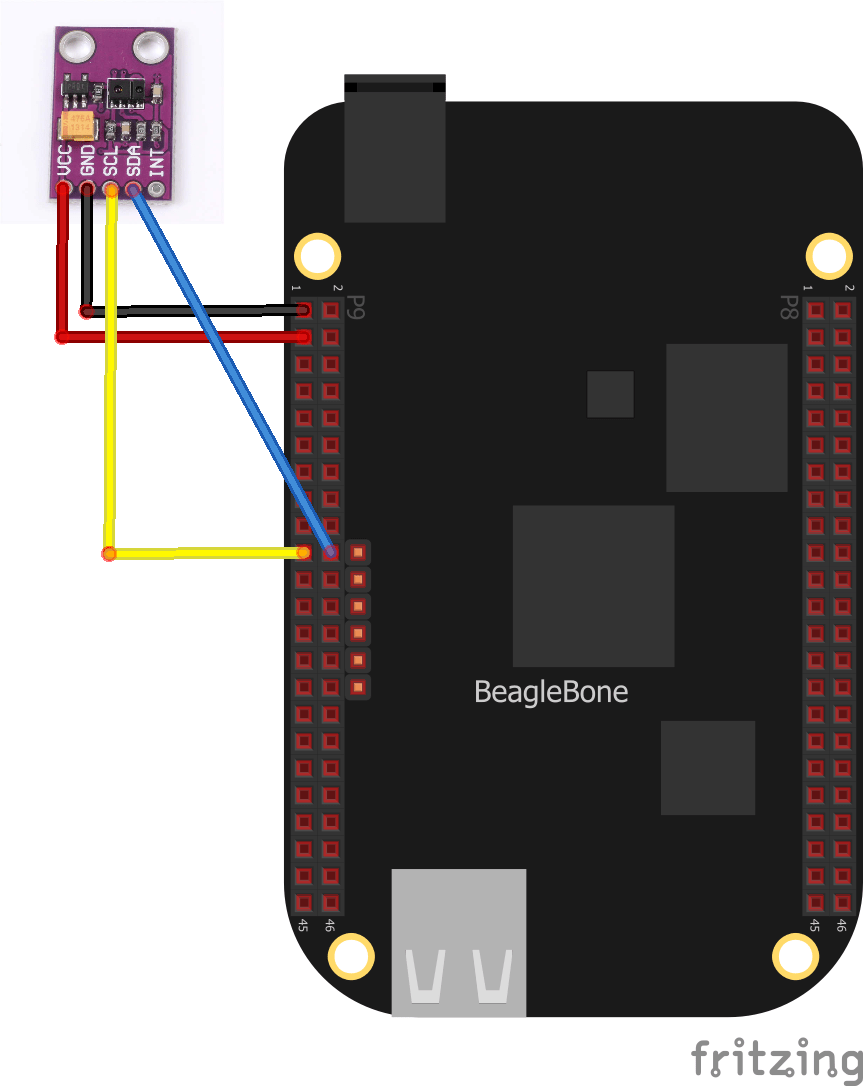
Code Example
Save this as TMD2771.py
[codesyntax lang=”python”]
import smbus import time # Get I2C bus bus = smbus.SMBus(2) # TMD2771 address, 0x39(57) # Select enable register 0x00(00), with command register, 0xA0(160) # 0x0F(15) Power ON, Wait enable, Proximity enable, ALS enable bus.write_byte_data(0x39, 0x00 | 0xA0, 0x0F) # TMD2771 address, 0x39(57) # Select ALS time register 0x01(01), with command register, 0xA0(160) # 0xDB(219) ATime - 101 ms bus.write_byte_data(0x39, 0x01 | 0xA0, 0xDB) # TMD2771 address, 0x39(57) # Select proximity ADC time register 0x02(02), with command register, 0xA0(160) # 0xFF(255) PTime - 2.72 ms bus.write_byte_data(0x39, 0x02 | 0xA0, 0xFF) # TMD2771 address, 0x39(57) # Select Wait time register 0x03(03), with command register, 0xA0(160) # 0xFF(255) WTime - 2.72 ms bus.write_byte_data(0x39, 0x03 | 0xA0, 0xFF) # TMD2771 address, 0x39(57) # Select pulse count register 0x0E(14), with command register, 0xA0(160) # 0x04(04) Pulse count = 4 bus.write_byte_data(0x39, 0x0E | 0xA0, 0x04) # TMD2771 address, 0x39(57) # Select control register 0x0F(15), with command register, 0xA0(160) # 0x20(32) 120 mA LED strength, Proximity uses CH1 diode # Proximity gain 1x, ALS gain 1x bus.write_byte_data(0x39, 0x0F | 0xA0, 0x20) time.sleep(0.5) # TMD2771 address, 0x39(57) # Read data back from 0x14(20), with command register, 0xA0(160), 6 bytes # c0Data LSB, c0Data MSB, c1Data LSB, c1Data MSB, Proximity LSB, Proximity MSB data = bus.read_i2c_block_data(0x39, 0x14 | 0xA0, 6) # Convert the data c0Data = data[1] * 256 + data[0] c1Data = data[3] * 256 + data[2] proximity = data[5] * 256 + data[4] luminance = 0.0 CPL = (101.0) / 24.0 luminance1 = ((1.00 * c0Data) - (2 * c1Data)) / CPL luminance2 = ((0.6 * c0Data) - (1.00 * c1Data)) / CPL if luminance1 > 0 and luminance2 > 0 : if luminance1 > luminance2 : luminance = luminance1 else : luminance = luminance2 # Output data to screen print "Ambient Light luminance : %.2f lux" %luminance print "Proximity of the Device : %.2f" %proximity
[/codesyntax]
Output
Run this example and you should see the following , cover the sensor and alter the light on it
debian@beaglebone:/var/lib/cloud9/$ python TMD2771.py
Ambient Light luminance : 98.85 lux
Proximity of the Device : 0.00
debian@beaglebone:/var/lib/cloud9/$ python TMD2771.py
Ambient Light luminance : 0.24 lux
Proximity of the Device : 1023.00
debian@beaglebone:/var/lib/cloud9/$ python TMD2771.py
Ambient Light luminance : 104.79 lux
Proximity of the Device : 6.00
debian@beaglebone:/var/lib/cloud9/iain$
Links