In this example we connect an ADS1115 analog-to-digital converters to a Beaglebone. The code example will be in C
Lets look at the ADS1115.
The ADS1115 is a precision, low-power, 16-bit, I2C-compatible, analog-to-digital converters (ADCs) offered in an ultra-small, leadless, X2QFN-10 package, and a VSSOP-10 package. The ADS1115 incorporates a low-drift voltage reference and an oscillator.
The ADS1115 also incorporate a programmable gain amplifier (PGA) and a digital comparator. These features, along with a wide operating supply range, make the ADS1115 well suited for power- and space-constrained, sensor measurement applications.
The ADS1115 performs conversions at data rates up to 860 samples per second (SPS). The PGA offers input ranges from ±256 mV to ±6.144 V, allowing precise large- and small-signal measurements. The ADS1115 features an input multiplexer (MUX) that allows two differential or four single-ended input measurements. Use the digital comparator in the ADS1115 for under- and overvoltage detection.
The ADS1115 operates in either continuous-conversion mode or single-shot mode. The devices are automatically powered down after one conversion in single-shot mode; therefore, power consumption is significantly reduced during idle periods.
Connection
In this example I had connected the sensor to the Beaglebone I2C2 SCL and SDA pins
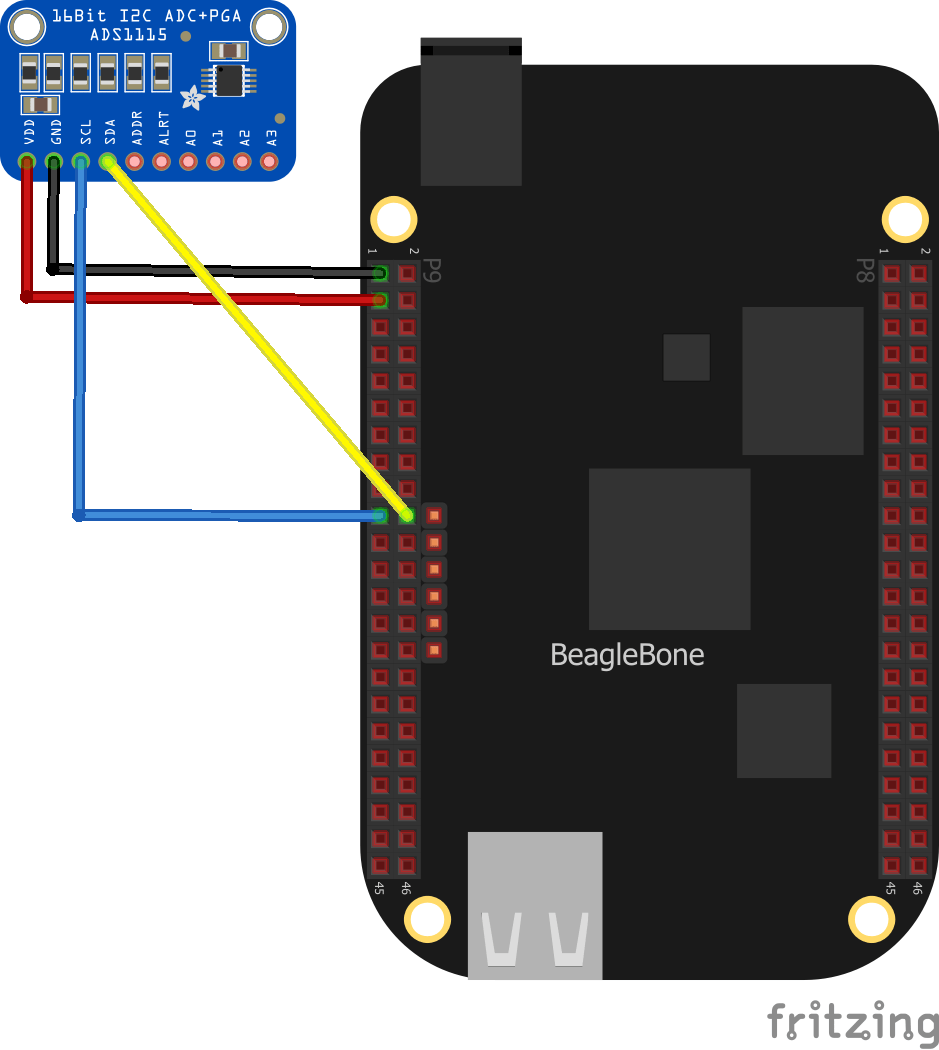
Parts List
Code
Save the following as ADS1115.c
[codesyntax lang=”cpp”]
#include <stdio.h> #include <stdlib.h> #include <linux/i2c-dev.h> #include <sys/ioctl.h> #include <fcntl.h> void main() { // Create I2C bus int file; char *bus = "/dev/i2c-2"; if ((file = open(bus, O_RDWR)) < 0) { printf("Failed to open the bus. \n"); exit(1); } // Get I2C device, ADS1115 I2C address is 0x48(72) ioctl(file, I2C_SLAVE, 0x48); // Select configuration register(0x01) // AINP = AIN0 and AINN = AIN1, +/- 2.048V // Continuous conversion mode, 128 SPS(0x84, 0x83) char config[3] = {0}; config[0] = 0x01; config[1] = 0x84; config[2] = 0x83; write(file, config, 3); sleep(1); // Read 2 bytes of data from register(0x00) // raw_adc msb, raw_adc lsb char reg[1] = {0x00}; write(file, reg, 1); char data[2]={0}; if(read(file, data, 2) != 2) { printf("Error : Input/Output Error \n"); } else { // Convert the data int raw_adc = (data[0] * 256 + data[1]); if (raw_adc > 32767) { raw_adc -= 65535; } // Output data to screen printf("Digital Value of Analog Input: %d \n", raw_adc); } }
[/codesyntax]
Output
Run the above example by opening a terminal and typing in
Compile the c program.
$>gcc ADS1115.c -o ADS1115
Run the c program.
$>./ADS1115
