In this article we will look at easily flashing the RGB LED and the 3 individual LEDs of the mxchip iot devkit,
You can see these on the image of the board below. There are 3 LEDs for Wi-Fi, Azure and User and we will have a simple example to flash these on and off
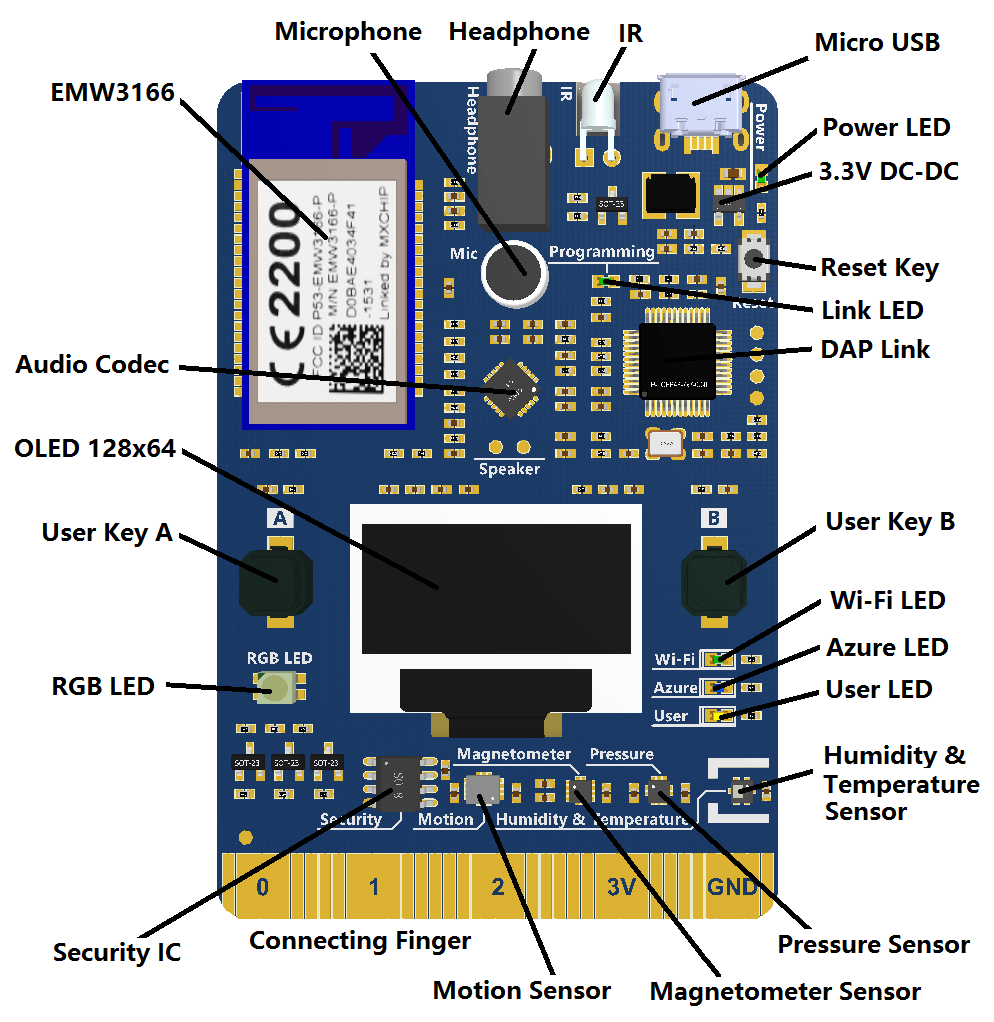
Parts
About $41 for the board
seeed studio MXChip AZ3166 IOT Developer Kit Compatible with Arduino
Code
This is for the Arduino IDE once you have added support for the MXChip
You could use the The RGB_LED class provides methods to set LED color and turn off LED but lets look at another way, each of the LEDs is defined and mapped in a file called PinNames.h which lies deep in your AZ3166 installation folder – here are the key parts
LED1 = MBED_GPIO_2,
LED2 = MBED_GPIO_9,
LED3 = MBED_GPIO_16,
LED_WIFI = LED1,
LED_AZURE = LED2,
LED_USER = LED3,
RGB_R = MBED_GPIO_31,
RGB_G = MBED_GPIO_27,
RGB_B = MBED_GPIO_12,
You can see that the RGB and 3 individual LEDs are clearly named and we can use these in our Arduino code.
Lets look at a couple of examples
First of all, the three individual LEDs, lets just switch these all on and off – a glorified blink sketch
[codesyntax lang=”cpp”]
void setup() { pinMode(LED_USER, OUTPUT); pinMode(LED_WIFI, OUTPUT); pinMode(LED_AZURE, OUTPUT); } void loop() { digitalWrite(LED_USER, HIGH); digitalWrite(LED_WIFI, HIGH); digitalWrite(LED_AZURE, HIGH); delay(500); digitalWrite(LED_USER, LOW); digitalWrite(LED_WIFI, LOW); digitalWrite(LED_AZURE, LOW); delay(500); }
[/codesyntax]
Now lets move onto the RGB LED
[codesyntax lang=”cpp”]
void setup() { // initialize the pins as digital output. pinMode(RGB_R, OUTPUT); pinMode(RGB_G, OUTPUT); pinMode(RGB_B, OUTPUT); } void loop() { //RGB is RED digitalWrite(RGB_R, HIGH); delay(1000); digitalWrite(RGB_R, LOW); delay(1000); // RGB is green digitalWrite(RGB_G, HIGH); delay(1000); digitalWrite(RGB_G, LOW); delay(1000); // RGB is blue digitalWrite(RGB_B, HIGH); delay(1000); digitalWrite(RGB_B, LOW); delay(1000); // you can mix leds digitalWrite(RGB_R, HIGH); digitalWrite(RGB_B, HIGH); delay(1000); digitalWrite(RGB_R, LOW); digitalWrite(RGB_B, LOW); delay(1000); digitalWrite(RGB_R, HIGH); digitalWrite(RGB_G, HIGH); delay(1000); digitalWrite(RGB_R, LOW); digitalWrite(RGB_G, LOW); delay(1000); }
[/codesyntax]