In this article we look at an MMA8452Q connected to a Beaglebone first of all lets look at the sensor.
Lets look at some information about the sensor
The MMA8452Q is a smart, low-power, three-axis, capacitive, micromachined accelerometer with 12 bits of resolution. This accelerometer is packed with embedded functions with flexible user programmable options, configurable to two interrupt pins. Embedded interrupt functions allow for overall power savings relieving the host processor from continuously polling data.
The MMA8452Q has user selectable full scales of ±2 g/±4 g/±8 g with high-pass filtered data as well as non-filtered data available real-time. The device can be configured to generate inertial wakeup interrupt signals from any combination of the configurable embedded functions allowing the MMA8452Q to monitor events and remain in a low-power mode during periods of inactivity
Parts Required
Name | Link |
Beaglebone | BeagleBone Black TI AM3358 Cortex-A8 development BB-Black Rev.C |
MMA8452Q | |
Connecting wire | Dupont line 120pcs 20cm male to male + male to female and female to female jumper wire |
Schematic/Connection
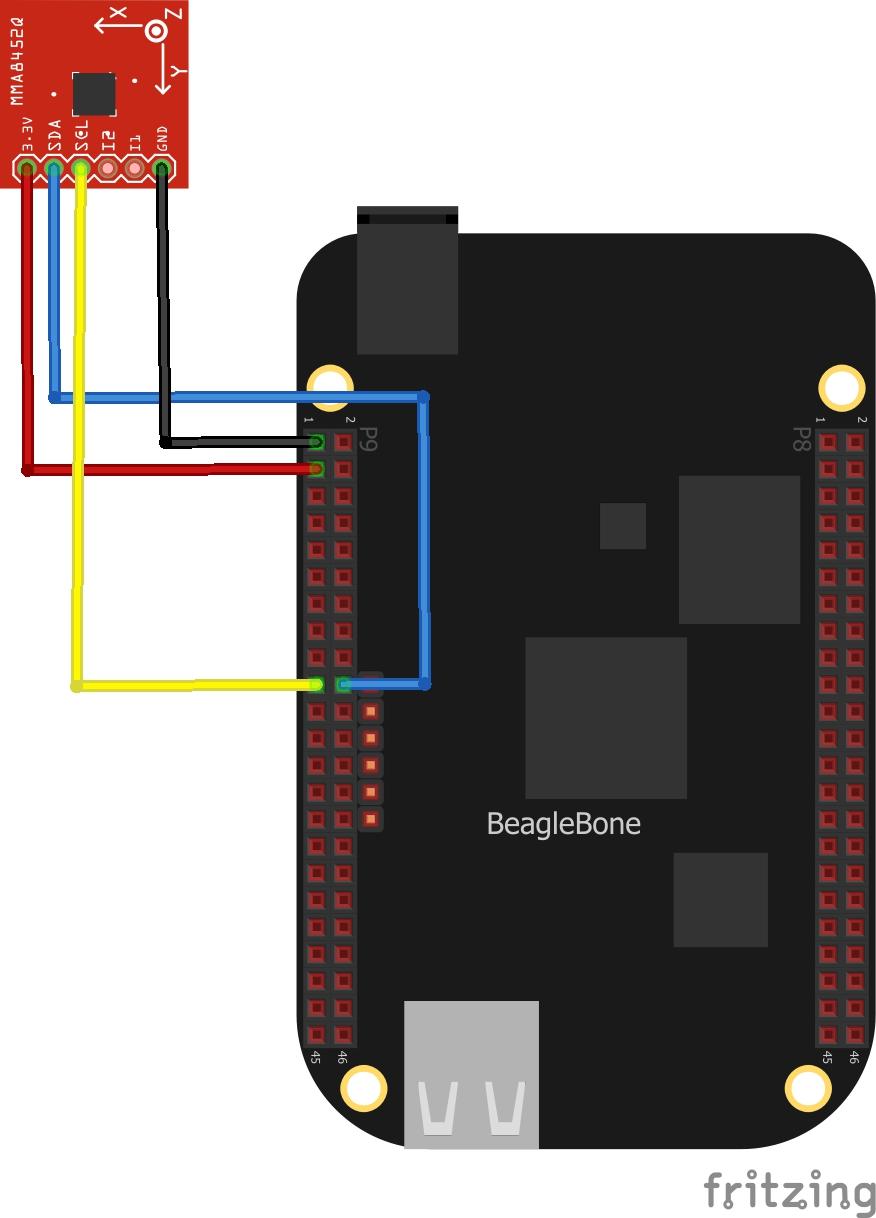
Code Example
This is a controleverything example – they have code examples for various platforms. This is the C example from https://github.com/ControlEverythingCommunity/MMA8452Q
[codesyntax lang=”cpp”]
#include <stdio.h> #include <stdlib.h> #include <linux/i2c-dev.h> #include <sys/ioctl.h> #include <fcntl.h> void main() { // Create I2C bus int file; char *bus = "/dev/i2c-2"; if((file = open(bus, O_RDWR)) < 0) { printf("Failed to open the bus. \n"); exit(1); } // Get I2C device, MMA8452Q I2C address is 0x1C(28) ioctl(file, I2C_SLAVE, 0x1C); // Select mode register(0x2A) // Standby mode(0x00) char config[2] = {0}; config[0] = 0x2A; config[1] = 0x00; write(file, config, 2); // Select mode register(0x2A) // Active mode(0x01) config[0] = 0x2A; config[1] = 0x01; write(file, config, 2); // Select configuration register(0x0E) // Set range to +/- 2g(0x00) config[0] = 0x0E; config[1] = 0x00; write(file, config, 2); sleep(0.5); // Read 7 bytes of data(0x00) // staus, xAccl msb, xAccl lsb, yAccl msb, yAccl lsb, zAccl msb, zAccl lsb char reg[1] = {0x00}; write(file, reg, 1); char data[7] = {0}; if(read(file, data, 7) != 7) { printf("Error : Input/Output error \n"); } else { // Convert the data to 12-bits int xAccl = ((data[1] * 256) + data[2]) / 16; if(xAccl > 2047) { xAccl -= 4096; } int yAccl = ((data[3] * 256) + data[4]) / 16; if(yAccl > 2047) { yAccl -= 4096; } int zAccl = ((data[5] * 256) + data[6]) / 16; if(zAccl > 2047) { zAccl -= 4096; } // Output data to screen printf("Acceleration in X-Axis : %d \n", xAccl); printf("Acceleration in Y-Axis : %d \n", yAccl); printf("Acceleration in Z-Axis : %d \n", zAccl); } }
[/codesyntax]
Save this as MMA8452Q.c, I used the Cloud 9 IDE
First of all compile the c program.
$>gcc MMA8452Q.c -o MMA8452Q
Run the c program.
$>./MMA8452Q
Output
After running you should see something like this
Acceleration in X-Axis : 467
Acceleration in Y-Axis : 568
Acceleration in Z-Axis : 723